How Developers Can Improve Their Debugging Techniques By Gustavo Woltmann
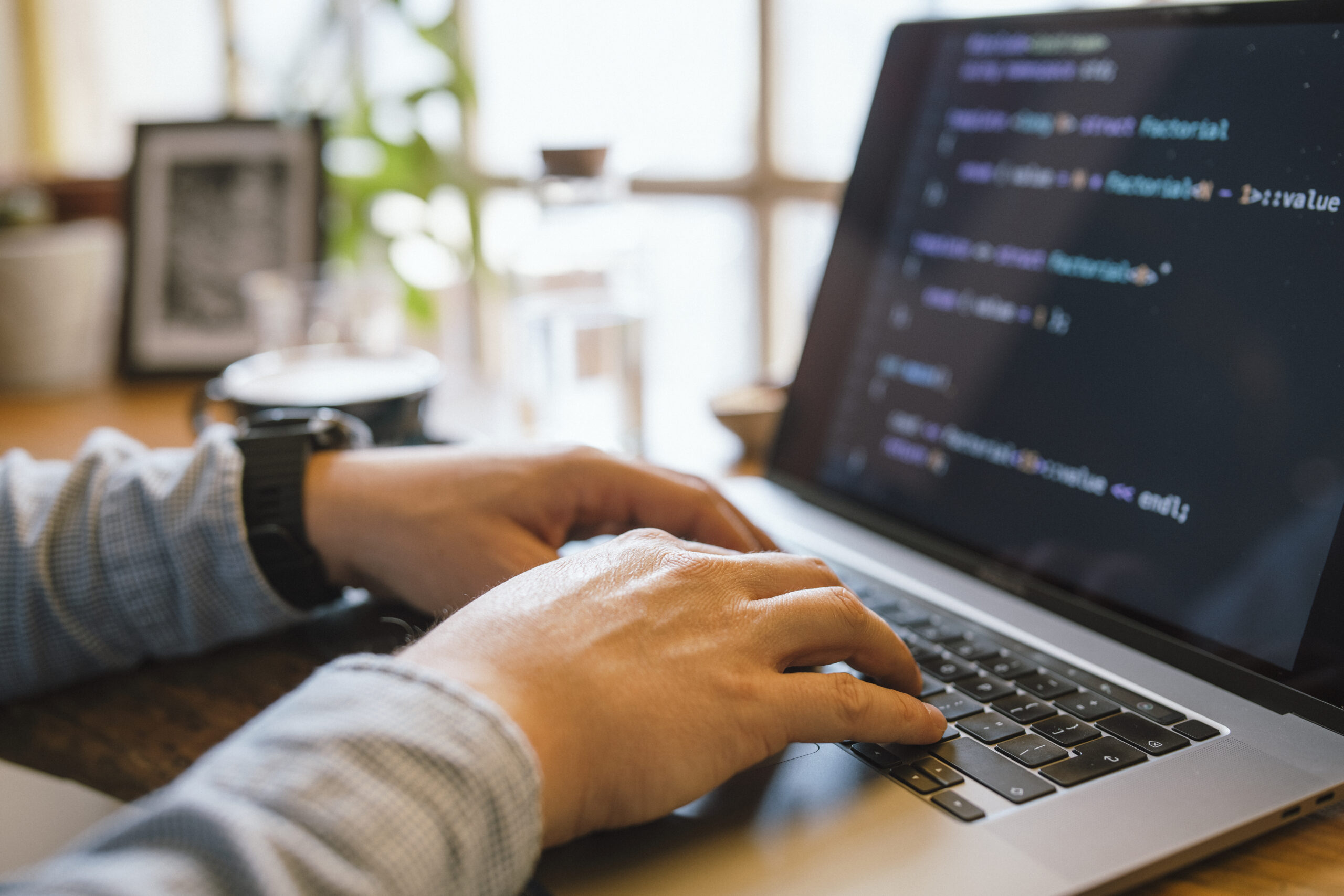
Debugging is One of the more critical — but typically forgotten — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to resolve difficulties proficiently. No matter if you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially increase your productiveness. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging expertise is by mastering the equipment they use daily. Whilst crafting code is a person Section of advancement, understanding how to connect with it efficiently throughout execution is Similarly critical. Modern day development environments appear equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these applications can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications let you set breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When applied appropriately, they let you observe precisely how your code behaves in the course of execution, which is priceless for monitoring down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-stop builders. They help you inspect the DOM, check community requests, check out serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can change discouraging UI problems into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with Edition Management units like Git to know code historical past, come across the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment making sure that when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time it is possible to commit fixing the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
The most essential — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request concerns like: What steps resulted in The difficulty? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise situations under which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the situation in your local ecosystem. This might necessarily mean inputting the identical info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, look at creating automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem can be atmosphere-distinct — it'd happen only on specific functioning systems, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires persistence, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. Having a reproducible scenario, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to looking at them as discouraging interruptions, developers must discover to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the message diligently As well as in complete. Lots of builders, especially when underneath time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read through and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to examine the context where the error transpired. Check out similar log entries, input values, and recent changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues more quickly, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true issues, and Lethal if the program can’t carry on.
Steer clear of flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Focus on vital functions, state improvements, input/output values, and important final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging strategy, you may reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers should strategy the method similar to a detective resolving a secret. This mindset will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What may very well be resulting in this habits? Have any adjustments not too long ago been produced towards the codebase? Has this issue happened in advance of underneath related situations? The objective is usually to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay back near attention to smaller specifics. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and client, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden problems in intricate devices.
Write Tests
Composing assessments is among the simplest tips on how to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security Internet that offers you assurance when earning changes to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely in which and when an issue occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can rapidly reveal whether a selected bit of logic is Performing as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that different parts of your software more info perform together effortlessly. They’re notably helpful for catching bugs that manifest in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Believe critically regarding your code. To test a element correctly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails persistently, you can give attention to repairing the bug and check out your check move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In brief, producing checks turns debugging from the irritating guessing recreation right into a structured and predictable system—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the situation—gazing your monitor for hours, attempting Remedy right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
If you're far too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear mistakes or misreading code which you wrote just hours previously. On this state, your brain becomes fewer economical at challenge-fixing. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of an issue when they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support stop burnout, especially through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
Should you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each bug you come across is much more than simply A short lived setback—It is an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial should you make time to replicate and review what went wrong.
Begin by asking oneself a number of essential issues when the bug is fixed: What prompted it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code opinions, or logging? The responses generally expose blind spots with your workflow or comprehension and allow you to Create more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see patterns—recurring issues or common issues—you can proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your friends is often Specially highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast information-sharing session, helping Many others stay away from the same challenge boosts crew efficiency and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your improvement journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but people that continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.