How Builders Can Increase Their Debugging Abilities By Gustavo Woltmann
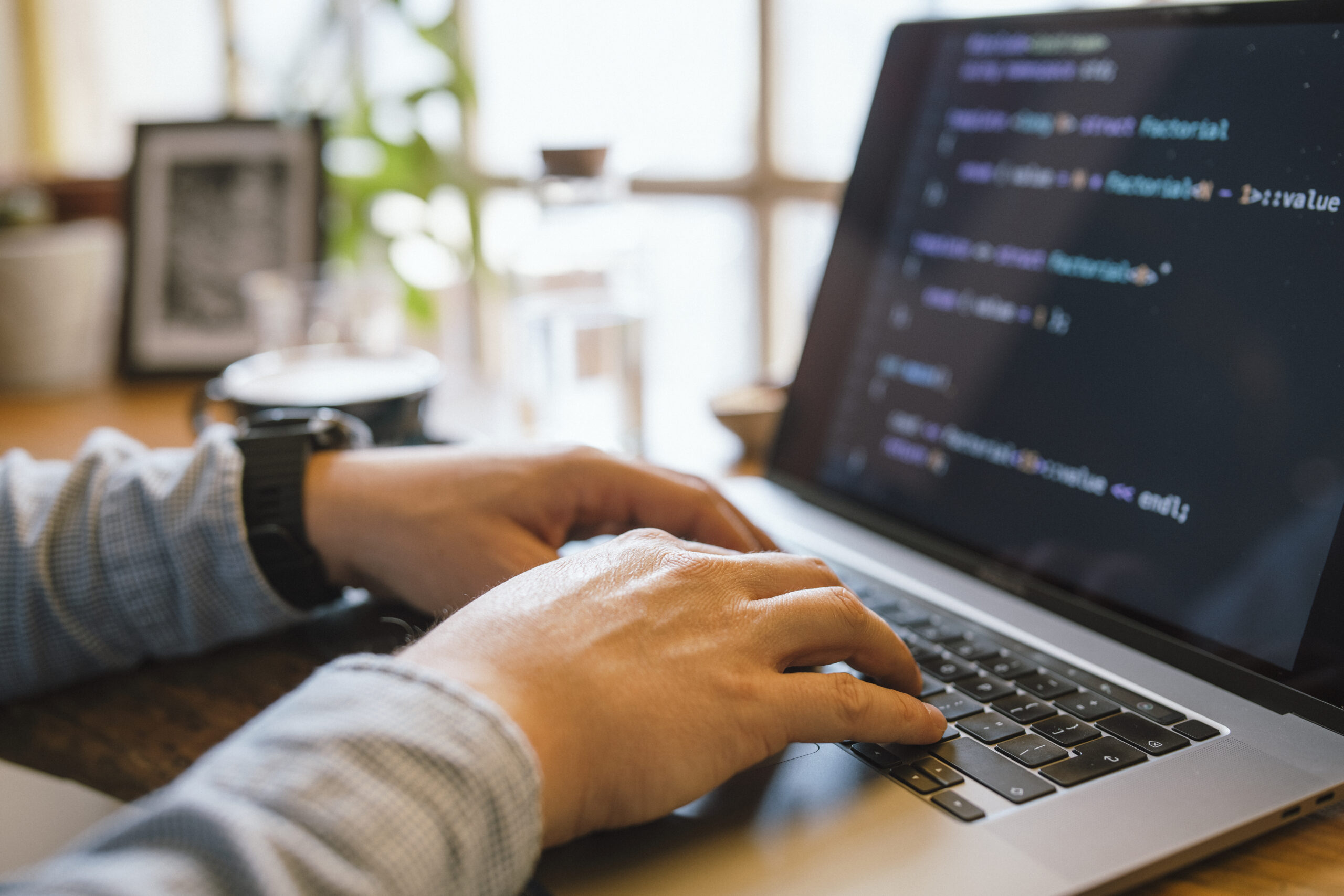
Debugging is Probably the most crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really nearly fixing broken code; it’s about comprehension how and why factors go Erroneous, and Discovering to Believe methodically to solve issues effectively. No matter whether you're a novice or a seasoned developer, sharpening your debugging expertise can conserve hrs of stress and substantially help your productiveness. Here's quite a few procedures that will help developers level up their debugging video game by me, Gustavo Woltmann.
Grasp Your Instruments
Among the list of fastest means builders can elevate their debugging capabilities is by mastering the instruments they use on a daily basis. Even though creating code is 1 part of development, understanding how to connect with it proficiently for the duration of execution is equally vital. Modern enhancement environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the floor of what these tools can perform.
Get, for example, an Built-in Improvement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, move as a result of code line by line, and in some cases modify code around the fly. When applied appropriately, they Permit you to observe accurately how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, such as Chrome DevTools, are indispensable for front-finish builders. They permit you to inspect the DOM, watch community requests, check out serious-time efficiency metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of operating processes and memory administration. Discovering these resources could have a steeper Discovering curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation control methods like Git to be familiar with code history, locate the exact minute bugs ended up released, and isolate problematic changes.
In the long run, mastering your tools indicates going past default options and shortcuts — it’s about building an intimate expertise in your development atmosphere to ensure that when concerns come up, you’re not dropped at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the particular challenge rather then fumbling as a result of the procedure.
Reproduce the situation
The most essential — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Just before jumping into the code or creating guesses, developers have to have to produce a steady surroundings or scenario where by the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as you can. Ask concerns like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug occurs.
When you finally’ve collected more than enough details, seek to recreate the challenge in your neighborhood setting. This might necessarily mean inputting precisely the same data, simulating related consumer interactions, or mimicking system states. If The problem appears intermittently, look at writing automated checks that replicate the edge situations or point out transitions included. These checks not just enable expose the issue and also prevent regressions Later on.
From time to time, The problem can be environment-certain — it would materialize only on particular working systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical strategy. But as soon as you can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible scenario, You should utilize your debugging applications more successfully, check prospective fixes securely, and talk additional Plainly with the staff or people. It turns an summary grievance into a concrete problem — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most respected clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders need to find out to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, where it took place, and often even why it happened — if you know the way to interpret them.
Get started by looking at the concept carefully and in full. Quite a few developers, especially when underneath time strain, glance at the main line and straight away start out producing assumptions. But further while in the error stack or logs may perhaps lie the real root result in. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it stage to a certain file and line quantity? What module or purpose activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and Discovering to recognize these can considerably speed up your debugging method.
Some errors are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the error happened. Examine relevant log entries, enter values, and up to date adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, helping you comprehend what’s taking place beneath the hood with no need to pause execution or stage throughout the code line by line.
A superb logging approach begins with realizing what to log and at what amount. Prevalent logging degrees incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic facts during development, Facts for normal functions (like productive commence-ups), WARN for opportunity difficulties that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and slow down your program. Concentrate on vital functions, state variations, enter/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how here variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging tactic, you can decrease the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a sort of investigation. To effectively determine and take care of bugs, developers should strategy the method similar to a detective resolving a secret. This mindset assists break down advanced issues into manageable parts and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the signs of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a clear image of what’s happening.
Next, type hypotheses. Inquire your self: What might be causing this actions? Have any improvements not long ago been manufactured for the codebase? Has this problem occurred prior to less than identical instances? The purpose is always to narrow down alternatives and discover prospective culprits.
Then, examination your theories systematically. Attempt to recreate the problem in a very controlled environment. For those who suspect a particular function or ingredient, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Pay out shut consideration to small details. Bugs generally hide during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of completely being familiar with it. Short-term fixes may well hide the true problem, just for it to resurface later.
And finally, continue to keep notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for foreseeable future challenges and assist Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and turn into more practical at uncovering concealed problems in elaborate methods.
Compose Assessments
Producing checks is one of the most effective approaches to increase your debugging techniques and overall improvement effectiveness. Assessments not simply assistance catch bugs early but additionally serve as a safety net that gives you self-assurance when producing alterations to your codebase. A well-tested application is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with device tests, which concentrate on person functions or modules. These little, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Any time a take a look at fails, you quickly know the place to search, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—problems that reappear after Beforehand staying fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that different areas of your application get the job done collectively smoothly. They’re specially valuable for catching bugs that happen in elaborate programs with numerous factors or providers interacting. If something breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This amount of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug could be a robust first step. After the exam fails constantly, you may concentrate on repairing the bug and enjoy your test move when The problem is fixed. This method makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture more bugs, more quickly and more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too extensive, cognitive exhaustion sets in. You would possibly start overlooking obvious faults or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-solving. A brief wander, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your focus. Many builders report obtaining the root of a problem after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance avert burnout, Specifically throughout longer debugging classes. Sitting before a display, mentally stuck, is not simply unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under limited deadlines, nevertheless it basically results in speedier and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial in case you make the effort to replicate and review what went Incorrect.
Begin by asking on your own a handful of key queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or comprehension and help you build stronger coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring issues or frequent errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast expertise-sharing session, aiding Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical elements of your enhancement journey. All things considered, a few of the finest developers are not the ones who write great code, but those that repeatedly learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you're knee-deep in the mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.